일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- macro lens #EF #FD
- Tarantula #3D 프린터
- Xeon #E5-2680
- Callaway #Mavrik #Epic Flash
- 매크로렌즈 #리버스링
- 다이슨 #배터리
- Laptop #CPUID
- x99 itx/ac
- Arduino #PlatformIO #macOS
- centos7 #yum update #/boot
- ESP32 #Arduino
- XTU #Virtual Machine System
- VMware #Shared Folder
- VNC #Firewall #CenOS7 #VMware
- k6 #피코프레소
- razer #deathadder #viper #g102
- 피코프레소 #ITOP40
- Dell #Latitude #BIOS
- fat32 #rufus
- VirtualBox #VMware
- CM-EF-NEX
- TensorFlow #Python #pip
- egpu #aorus gaming box #gtx1070 #tb3
- Arduino #Wall Plotter
- Oh My Zsh #macOS
- Linux #VirtualBox
- Octave #homebrew #macOS
- cycloidal #rv reducer
- ITOP40
- Java #MacBook #macOS
- Today
- Total
목록IT/비트코인 (4)
얕고 넓게
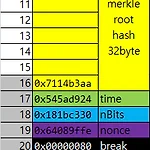
2025.02.04모두 정리가 되었지만 Byte Order 가 이해가 안돼서 다시 확인.현재 코드기준version + previous hash + root hash + ...각 아이템은 보통의 Big Endian 이지만 전체로 보면 Little Endian이 되어야 한다.string msg = “00112233…”message[0] = 0x00;message[1] = 0x11;message[2] = 0x22;message[3] = 0x33;sha256_top에서 1byte 데이터를 4byte로 만들 때 Little로 바꾼다.UINT msg = 0x00112233;sha256_core에서 BIG_ENDIAN이 선언되어 있지 않기 때문에 Little을 다시 Big으로 바꾼다.#if defined(BIG_E..
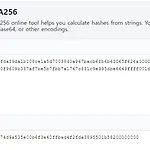
2025.01.09nonce가 자동으로 증가하도록 코드를 수정하다 보니 이제까지 의 예제 string이 뭔가 잘못되어 있다.모든 정보가 string으로 된다고 했는데 다시 정리해서 보니 말이 안된다.일단 다시 정리해더는 4+32+32+4+4+4 => 80byte (640bit)Hash가 00000000000000000024fb37364cbf81fd49cc2d51c09c75c35433c3a1945d04 인데스트링으로 그대로 입력하면 64 char 되므로 32byte가 아니다.보이는 것만 hex formathttps://www.blockchain.com/explorer/blocks/btc/500000#500000 자료로 다시 테스트Version 0x20000000Preve(#499999) 0000000000..
2025.01.02KISA의 SHA256을 다시 테스트 하다보니 무언가 이상해서 다시 정리.https://seed.kisa.or.kr/kisa/Board/21/detailView.do gcc는 warnig, g++ 로 컴파일 하면 에러 발생invalid conversion from 'UINT* {aka unsigned int*}' to 'ULONG_PTR {aka long unsigned int*}' [-fpermissive]typedef unsigned long ULONG;typedef ULONG* ULONG_PTR;void SHA256_Transform(ULONG_PTR Message, ULONG_PTR ChainVar) SHA256_Transform((ULONG_PTR)Info->sz..
2022.02.050. Ref.https://www.youtube.com/watch?v=JM3j7uBKnt8 https://www.analyticsvidhya.com/blog/2021/05/how-to-mine-bitcoin-using-python-part-i/ How to mine Bitcoin using Python? ( Part - I ) - Analytics VidhyaBitcoin ecosystem is made out of nodes or miners who execute the bitcoin code and store it in the blockchain. Lets mine bitcoin using pythonwww.analyticsvidhya.comhttps://blog.naver.com..