Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | 7 |
8 | 9 | 10 | 11 | 12 | 13 | 14 |
15 | 16 | 17 | 18 | 19 | 20 | 21 |
22 | 23 | 24 | 25 | 26 | 27 | 28 |
29 | 30 |
Tags
- VirtualBox #VMware
- XTU #Virtual Machine System
- x99 itx/ac
- 피코프레소 #ITOP40
- Laptop #CPUID
- fat32 #rufus
- Arduino #Wall Plotter
- Callaway #Mavrik #Epic Flash
- 매크로렌즈 #리버스링
- Tarantula #3D 프린터
- k6 #피코프레소
- VNC #Firewall #CenOS7 #VMware
- Xeon #E5-2680
- Dell #Latitude #BIOS
- TensorFlow #Python #pip
- centos7 #yum update #/boot
- Octave #homebrew #macOS
- 다이슨 #배터리
- macro lens #EF #FD
- Arduino #PlatformIO #macOS
- Java #MacBook #macOS
- Linux #VirtualBox
- ESP32 #Arduino
- VMware #Shared Folder
- CM-EF-NEX
- egpu #aorus gaming box #gtx1070 #tb3
- Oh My Zsh #macOS
- razer #deathadder #viper #g102
- cycloidal #rv reducer
- ITOP40
Archives
- Today
- Total
얕고 넓게
[AI] Llama 파인튜닝 with Copilot 본문
2025.04.08
지난 번 이해 못한 파인튜닝을 다시 도전.
다른 블로그에서 학습용 데이터 만드는 것부터 이상했는데,
Copilot에게 물어서 정리했다.
QnA형태로 구성된 test.txt 파일을 이용하여 로컬에 있는 aa.gguf 모델 파인 튜닝하는 순서와 구체적인 파이썬 코드를 알려줘
# Step 1: 환경 설정
import transformers
import torch
# 필요한 라이브러리 설치
!pip install transformers datasets
# Step 2: 데이터 준비
def load_data(file_path):
with open(file_path, 'r', encoding='utf-8') as f:
lines = f.readlines()
data = [{"question": line.split('Q:')[1].strip(),
"answer": line.split('A:')[1].strip()} for line in lines if 'Q:' in line and 'A:' in line]
return data
data = load_data("test.txt")
# Step 3: 모델 로드
from transformers import AutoModelForCausalLM, AutoTokenizer
model_path = "aa.gguf" # 로컬 모델 경로
model = AutoModelForCausalLM.from_pretrained(model_path)
tokenizer = AutoTokenizer.from_pretrained(model_path)
# Step 4: 파인 튜닝 수행
from transformers import Trainer, TrainingArguments
training_args = TrainingArguments(
output_dir="./fine_tuned_model",
num_train_epochs=3,
per_device_train_batch_size=8,
save_steps=500,
save_total_limit=2,
logging_dir='./logs',
)
trainer = Trainer(
model=model,
args=training_args,
train_dataset=data,
)
trainer.train()
# Step 5: 모델 평가
results = trainer.evaluate()
# Step 6: 모델 저장
model.save_pretrained("./fine_tuned_model")
tokenizer.save_pretrained("./fine_tuned_model")
LoRA, PEFT 를 적용해줘.
# Step 1: 환경 설정
import torch
from transformers import AutoTokenizer, AutoModelForCausalLM
from peft import PeftConfig, PeftModel, PeftModelForCausalLM, TaskType, LoraConfig, get_peft_model
# 필요한 라이브러리 설치
!pip install transformers peft
# Step 2: 데이터 준비
from datasets import load_dataset
# 텍스트 파일 데이터 파싱
def load_txt_data(file_path):
data = []
with open(file_path, 'r', encoding='utf-8') as f:
for line in f:
if 'Q:' in line and 'A:' in line:
question = line.split('Q:')[1].split('A:')[0].strip()
answer = line.split('A:')[1].strip()
data.append({"question": question, "answer": answer})
return data
# test.txt 파일 데이터 로드
#data = load_txt_data("test.txt")
print(data) # 데이터를 확인
# QnA 데이터셋 로드 (예: test.txt -> .json 변환 가정)
dataset = load_dataset("json", data_files={"train": "test.json"})
# Step 3: 모델 및 토크나이저 로드
base_model_path = "aa.gguf" # 로컬 경로 설정
tokenizer = AutoTokenizer.from_pretrained(base_model_path)
model = AutoModelForCausalLM.from_pretrained(base_model_path)
# Step 4: PEFT와 LoRA 적용
# LoRA 설정
lora_config = LoraConfig(
task_type=TaskType.CAUSAL_LM,
r=8, # 랭크 설정 (메모리 최적화)
lora_alpha=16, # LoRA 스케일링 팩터
lora_dropout=0.1, # 드롭아웃
target_modules=["query", "value"] # 특정 모듈에만 적용
)
# PEFT 모델 적용
peft_model = get_peft_model(model, lora_config)
# Step 5: RoLA 적용 (정규화)
# RoLA를 적용하기 위해 정규화 관련 Loss 함수 설정 (가정)
def r2_reg_loss(model, inputs, alpha=0.01):
"""간단한 RoLA Regularization Loss 적용 예제"""
outputs = model(**inputs)
logits = outputs.logits
reg_loss = alpha * torch.norm(logits, p=2) # L2 정규화
return outputs.loss + reg_loss
# Step 6: 모델 학습 준비
from transformers import TrainingArguments, Trainer
training_args = TrainingArguments(
output_dir="./peft_rola_model",
learning_rate=5e-5,
num_train_epochs=3,
per_device_train_batch_size=8,
save_steps=500,
save_total_limit=2,
logging_dir="./logs",
logging_steps=10,
evaluation_strategy="epoch",
)
# Trainer 설정
trainer = Trainer(
model=peft_model,
args=training_args,
train_dataset=dataset["train"],
tokenizer=tokenizer,
compute_loss=r2_reg_loss # RoLA 적용
)
# Step 7: 모델 학습 수행
trainer.train()
# Step 8: 결과 평가 및 저장
trainer.save_model("./peft_rola_model")
tokenizer.save_pretrained("./peft_rola_model")
# Step 1: 환경 설정
import torch
from transformers import AutoTokenizer, AutoModelForCausalLM
from peft import LoraConfig, get_peft_model
# 필요한 라이브러리 설치
!pip install transformers peft
# Step 2: 텍스트 데이터 로드 및 처리
def load_txt_data(file_path):
data = []
with open(file_path, 'r', encoding='utf-8') as f:
for line in f:
if 'Q:' in line and 'A:' in line:
question = line.split('Q:')[1].split('A:')[0].strip()
answer = line.split('A:')[1].strip()
data.append({"question": question, "answer": answer})
return data
# test.txt 파일 데이터 로드
file_path = "test.txt"
data = load_txt_data(file_path)
# 질문-답변 데이터를 처리하여 학습 데이터셋 생성
from datasets import Dataset
train_data = Dataset.from_dict({"input": [item["question"] for item in data],
"output": [item["answer"] for item in data]})
# Step 3: 모델 및 토크나이저 로드
base_model_path = "aa.gguf" # 로컬 모델 경로 설정
tokenizer = AutoTokenizer.from_pretrained(base_model_path)
model = AutoModelForCausalLM.from_pretrained(base_model_path)
# Step 4: PEFT와 LoRA 적용
# LoRA 설정
lora_config = LoraConfig(
task_type="CAUSAL_LM",
r=8, # 랭크 설정
lora_alpha=16, # LoRA 스케일링 팩터
lora_dropout=0.1, # 드롭아웃 비율
target_modules=["query", "value"], # 특정 모듈에만 적용
)
# PEFT 모델 적용
peft_model = get_peft_model(model, lora_config)
# Step 5: RoLA 적용 (정규화)
# RoLA를 적용하는 Loss 함수 정의
def r2_reg_loss(model, inputs, alpha=0.01):
"""RoLA Regularization Loss"""
outputs = model(**inputs)
logits = outputs.logits
reg_loss = alpha * torch.norm(logits, p=2) # L2 정규화 추가
return outputs.loss + reg_loss
# Step 6: 모델 학습 준비
from transformers import Trainer, TrainingArguments
training_args = TrainingArguments(
output_dir="./peft_rola_model",
learning_rate=5e-5,
num_train_epochs=3,
per_device_train_batch_size=8,
save_steps=500,
save_total_limit=2,
logging_dir="./logs",
evaluation_strategy="epoch",
)
trainer = Trainer(
model=peft_model,
args=training_args,
train_dataset=train_data,
tokenizer=tokenizer,
compute_loss=r2_reg_loss # RoLA 적용
)
# Step 7: 모델 학습 수행
trainer.train()
# Step 8: 결과 평가 및 저장
trainer.save_model("./peft_rola_model")
tokenizer.save_pretrained("./peft_rola_model")
모델파일 다운로드 받기가 안된다.
허깅페이스
https://huggingface.co/meta-llama/Llama-3.1-8B/tree/main
meta-llama/Llama-3.1-8B at main
The information you provide will be collected, stored, processed and shared in accordance with the Meta Privacy Policy. LLAMA 3.1 COMMUNITY LICENSE AGREEMENT Llama 3.1 Version Release Date: July 23, 2024 "Agreement" means the terms and conditions for use,
huggingface.co
*.safetensors 만 있다.
파인튜닝에 사용가능하다고 함. ollama는 gguf만 가능
그냥은 다운로드가 안된다.
Meta
다운로드 위에서 정보 입력
파이썬 관련 툴을 설치 후 진행
You are all set!
Request ID: 1413*************
Requested models:
- Llama 3.1: 8B & 405B
The models listed below are now available to you under the terms of the Llama community license agreement. By downloading a model, you are agreeing to the terms and conditions of the License, Acceptable Use Policy and Meta’s privacy policy.
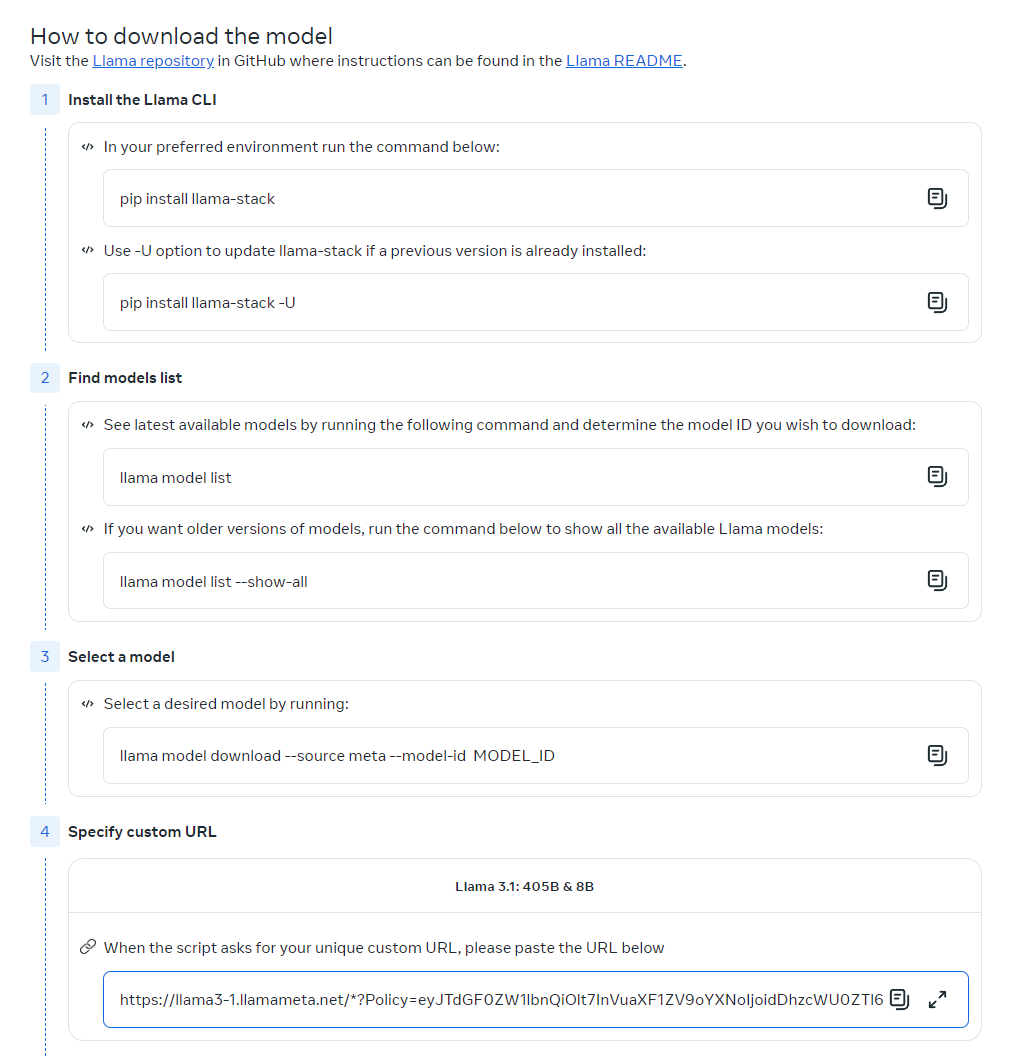
복사해서 붙여 넣으면 된다
Please save copies of the unique custom URLs provided above, they will remain valid for 48 hours to download each model up to 5 times, and requests can be submitted multiple times. An email with the download instructions will also be sent to the email address you used to request the models.
'IT > AI.ML' 카테고리의 다른 글
[AI] Llama 파인튜닝 @Ubuntu (0) | 2025.04.10 |
---|---|
[AI] 환경설정 @Ubuntu (0) | 2025.04.09 |
[AI] Open-WebUI: Docker 설치, 지우기, 다시... @Ubuntu (0) | 2025.04.03 |
[AI] Open-WebUI: Docker 설치 @Windows11 (0) | 2025.04.01 |
[AI] Open-WebUI Development Guide (0) | 2025.03.21 |